CSRF – Cross Site Request Forgery is a certain type of cyber attack, specifically when using cookies!
A different website would post content into a different domain when the user of the other domain is logged in or in certain other scenarios. CSRF is considered one of the major vulnerabilities and has been in the OWASP top 10 – Cross Site Request Forgery (CSRF).
If you are using token based authentication and if the token is stored in browser’s local storage, CSRF isn’t an issue. This is specifically when using cookies.
Basic Usage:
In .cshtml of web pages inside forms add the following tag:
@Html.AntiForgeryToken()
The above code fragment would render a hidden input element with a long random string.
In the controller class, decorate the action method with the following attribute:
[ValidateAntiForgeryToken]
When the action method is invoked, the validation happens. If the validation succeeds, the action method get invoked. If the validation fails, the action method does not get invoked.
Recommended Usages:
If we forget decorating a post method with [ValidateAntiForgeryToken], we would be susceptible to CSRF attack. Instead we can use a MiddleWare and use the Middleware in the Startup.cs
builder.Services.AddControllersWithViews((options) =>
{
options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute());
});
// or
builder.Services.AddMvc((options) =>
{
options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute());
});
There are other ways of customizing the middleware, for example if there is a use-case where json data is being sent to a web api and cookies are used for authentication, we can add a customizable header i.e in the calling code we would add the hidden element’s value as header and then make the call.
builder.Services.AddAntiforgery(options =>
{
options.HeaderName = "X-CSRF-TOKEN-HEADERNAME";
});
–
Mr. Kanti Kalyan Arumilli
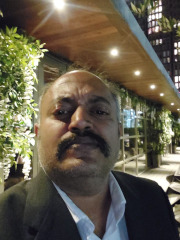
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.