I am NOT DBA, but by reading and following the instructions provided in some blog articles, I was able to use MariaDB Galera cluster.
My SaaS Product WebVeta, was running on MariaDB and then there was a error and had been a headache for over past 3 days.
I played around with MariaDB Galera over the past 2 days and here is what I think might be useful. This blog post is for developers or other non-DBA’s who want to quickly play around with MariaDB Galera, for example, one person startup founders like me in early stages and didn’t hire yet.
I had issues when I simulated crashes and recovering from crashes using MariaDB Galera if used in a multi-master write scenarios. Instead using one of the nodes for writing and the other nodes for reads seemed easier and can be recovered using some code / scripts. Sample code / scripts part gets discussed in this blog post.
Installation can be performed using any of these blog posts:
https://www.linode.com/docs/guides/how-to-set-up-mariadb-galera-clusters-on-ubuntu-2204
Based on internet blog posts, recovering from a crash means finding the node that had the most recent write and using that node as primary, but that seemed a little messy.
This is what I did and learned over the past 2 days of playing around with MariaDB Galera (as mentioned this blog post for non-DBA’s who want to use MariaDB Galera easily, for example, one person startup founders like me in early stages and didn’t hire yet):
I was writing into node-1. This way no need of messing with or trying to find latest write and then promoting as new master node. Because the same node is always the master.
If node-1 goes down, edit
/var/lib/mysql/grastate.dat
and modify the line
safe_to_bootstrap: 1
to have value of 1. Then
sudo galera_new_cluster
on node-1. Then restart all mariadb service on all other nodes.
sudo systemctl restart mariadb
This removes all the confusion and headache. However remember whenever node-1 goes down or reboots, this becomes a mess and the above steps need to be done.
I have some code that performs background tasks written in C# as part of my SaaS product – WebVeta.
I plan to add some extra functionality for 2nd, 3rd nodes to listen for restart mariadb service commands. The 1st nodes code would parse the galera.cnf figure out the clients, update grastate.dat, start node-1, look at cluster size, call the 2nd, 3rd nodes for restart.
That’s all for now, until I get a part-time / full-time DBA into my startup’s team.
Then why MariaDB Galera if not using multi-master? Later, when I hire a DBA, it’s easy to start using multi-master.
Azure: I hope Microsoft Azure offers MariaDB Galera as part of Azure Database services.
MariaDb Galera: MariaDB Galera team should consider using some kind of protocol for easily self-promoting of secondary nodes by using some kind of internal voting and easily adding nodes i.e if one node connects to another node, the new node gets information about rest of the nodes in the cluster and communicates with every other node.
–
Mr. Kanti Kalyan Arumilli
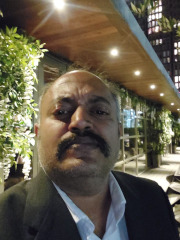
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.