Symmetric encryption is an encryption technique where the same set of keys are used for encryption and decryption. Whereas, Asymmetric encryption uses different keys i.e public key for encryption and associated private key for decryption.
TripleDES is an algorithm for implementing symmetric encryption.
TripleDES uses Key and IV.
public string EncryptTripleDES(string plainText, byte[] Key, byte[] IV)
{
byte[] encrypted;
using (TripleDESCryptoServiceProvider tdes = new TripleDESCryptoServiceProvider())
{
ICryptoTransform encryptor = tdes.CreateEncryptor(Key, IV);
using (MemoryStream ms = new MemoryStream())
{
using (CryptoStream cs = new CryptoStream(ms, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter sw = new StreamWriter(cs))
sw.Write(plainText);
encrypted = ms.ToArray();
}
}
}
return Convert.ToBase64String(encrypted);
}
The above code snippet is for encryption.
public string DecryptTripleDES(string cipherText, byte[] Key, byte[] IV)
{
string plaintext = null;
var cipherBytes = Convert.FromBase64String(cipherText);
using (TripleDESCryptoServiceProvider tdes = new TripleDESCryptoServiceProvider())
{
ICryptoTransform decryptor = tdes.CreateDecryptor(Key, IV);
using (MemoryStream ms = new MemoryStream(cipherBytes))
{
using (CryptoStream cs = new CryptoStream(ms, decryptor, CryptoStreamMode.Read))
{
using (StreamReader reader = new StreamReader(cs))
plaintext = reader.ReadToEnd();
}
}
}
return plaintext;
}
The above code snippet is for Decryption.
My open-source tool LightKeysTransfer uses TripleDES and the accompanying source code can be found at:
https://github.com/ALightTechnologyAndServicesLimited/LightKeysTransfer
The encryption/decryption related code can be found at:
–
Mr. Kanti Kalyan Arumilli
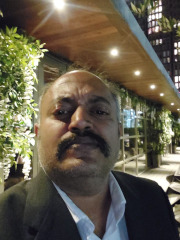
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.