gRPC is a bery efficient form of communication between different servers.
In the past each programming language had its own technique of Remote Invocation using a very efficient binary serialization and de-serialization. But then these services are not compatible across programming languages. Then webservices standards such as SOAP based on XML, REST API’s based on XML or JSON have evolved. SOAP and REST API’s are a standard and can be implemented in different programming languages. Even now, REST based API’s are the most popular choice. Now comes gRPC alleviating the problems and offers very high performance, development tools are shared across different programming languages and takes advantage of HTTP/2, HTTP/3 where possible.
gRPC re-uses server connections and offers significant advantages in terms of performance i.e the overhead of establishing and disconnecting connections gets minified. Efficient serialization and de-serialization offers high performance in terms of payload and speed i.e fewer CPU cycles and fewer network bytes.
The following tips are for .Net platform:
- Increase connection concurrency limit i.e by default the concurrency limit is 100, on large servers with many connections, if you see a performance hit i.e gRPC calls getting queued, consider increasing the concurrency limit.
- Enable SocketsHttpHandler.EnableMultipleHttp2Connections = true;
- Consider client side load balancing where applicable, server side load balancing adds a little extra latency, because the request reaches the load balancer and then gets routed to a server. With client side load balancing the client knows how to communicate with the different servers and sends requests appropriately removing the overhead of extra latency.
- If the service’s gRPC messages are larger than 96kb consider increasing the InitialConnectionWindowSize and InitialStreamWindowSize.
Latest versions of .Net support creating REST based API’s and gRPC based services with the same code – a topic for a different blog post, but definitely a reason to start using gRPC.
–
Mr. Kanti Kalyan Arumilli
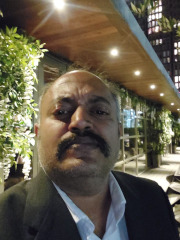
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.