In React Functional components, content can be shown or hidden based on conditions.
function Component1 {
return (
<React.Fragment>
<p>Hello!</p>
</React.Fragment>
);
}
The above component would always show the paragraph with the text “Hello!”. Now, let’s say if we are displaying content conditionally, we can use the following syntax:
function Component1 {
const [condition, setCondition] = React.useState(false);
return (
<React.Fragment>
{condition && (
<React.Fragment>
<p>Condition is true!</p>
</React.Fragment>
)}
{!condition && (
<React.Fragment>
<p>Condition is false!</p>
</React.Fragment>
)}
<p>Hello!</p>
</React.Fragment>
);
}
In the above code snippet, we have created a state variable condition and initialized to false. If condition is true, the paragraph with text – “Condition is true!” would be displayed. If condition is false, the paragraph with text – “Condition is false!” would be displayed. Irrespective of the value of condition, the paragraph with the text – “Hello!” would be displayed.
Based upon the application, the value of condition can be changed and the application’s user interface would be updated appropriately.
setCondition(true);
The above coding pattern can be used for example for displaying a “Please wait” message while some data is loading. Or showing a table only when there is data, else showing something like “No data!”
React can be progressively integrated into existing websites or for new websites, very easy learning curve and can be run from browser without even having the node based server-side rendering. Of course, node based server-side rendering etc… can be done like other SPA frameworks.
The production version of React in-browser scripts are ~132kb and about ~45.1kb compressed and can be used from CDNs. The custom JSX code can be transpiled before production deployment for slightly faster performance without using babel in browser.
This would be the content of a future post. In the post, I would discuss about transpiling and minifying.
Compressing and serving with gzip / brotli or even using your own CDN’s in the cloud is an entirely different topic, but can be done easily for static content.
–
Mr. Kanti Kalyan Arumilli
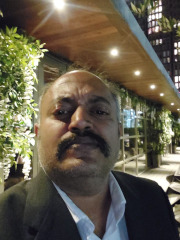
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.