Hash algorithms take some data and create fixed size representation. The representations can be of various sizes such as 32/64/128/256/512 bytes.
Hash generated are in-general non-reversible i.e you can’t get the original data from hash.
Cryptographic hashes use some high level of cryptography and should be used for sensitive information such as passwords etc…
Non-cryptographic hash implementations can be used for general information.
SHA256, SHA384 and SHA512 are for cryptographic hashes.
The above mentioned SHA implementations are secure but little slower.
Here are some faster hash implementations for non-secure data purposes:
FastHash – Can generate 32/64/128 bit hashes.
var sometext = "SOME TEXT";
ReadOnlySpan<byte> data = new ReadOnlySpan<byte>(Encoding.UTF8.GetBytes(sometext));
var retVal = FastHash.HashGenerator.GenerateHash128(data);
var guid = retVal.AsGuid().ToString();
OR
Convert.ToBase64String(retVal.AsSpan());
MurmurHash3 – Generates 128 bit hash and faster than FastHash’s 128 bit implementation.
var sometext = "SOME TEXT";
var data = Encoding.UTF8.GetBytes(sometext);
var retVal = murmurHasher.ComputeHash(data);
var guid = Convert.ToBase64String(retVal);
BLAKE2 – Generates 1-64 bytes i.e 8-512 bits hash.
var sometext = "SOME TEXT";
var bytes2 = Blake2Fast.Blake2b.ComputeHash(new ReadOnlySpan<byte>(Encoding.UTF8.GetBytes(sometext)));
var guid = Convert.ToBase64String(bytes2);
For specifying the hash-size in bytes use the overload for ComputeHash.
var bytes2 = Blake2Fast.Blake2b.ComputeHash(new ReadOnlySpan<byte>(<bytes>, Encoding.UTF8.GetBytes(sometext)));
The value of <bytes> can be between 1 and 64.
–
Mr. Kanti Kalyan Arumilli
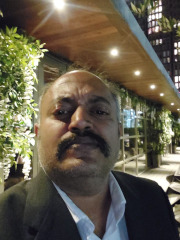
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.