Polly is a .Net library for writing resilient .Net code. The library can be found at: https://github.com/App-vNext/Polly.
Most of yesterday and this morning, I have been playing around with this library and found the library very useful.
When we develop modern web applications, we consume various services such as internal or 3rd party REST / SOAP / gRPC services. Sometimes, the 3rd party service might be intermittently unavailable. Polly allows a fluid way of re-writing resilient code.
There are several different policies available in Polly, but I liked Retry, Timeout, RateLimit, Cache, PolicyWrap.
The extensive documentation is very well-written and thanks to the 70 contributors who have put in effort for making a perfectly useful library and extensive documentation.
The retry policy, allows retrying multiple times, we can even specify how many times to retry, how long to wait before each retry etc…
Getting started code:
var retryThricePolicy = Policy.Handle<Exception>().Retry(2);
retryThricePolicy.Execute(() => {
DoSomething()
}
);
In the above code, we defined a policy to retry two more times if an Exception is thrown, we are not introducing any delay – not recommended for Production, because we want to use Exponential Backoff strategy.
The next line of code we are using the policy to execute the method DoSomething. In reality, we can have few more lines of code.
For introducing delay instead of Retry, we can use WaitAndRetry().
Timeout() is for handling timeouts.
Cache() for write-through caching strategy.
RateLimit for handling rate limits i.e x number of request per second or per minute etc…
PolicyWrap for a combination of policies.
Carefully by observing and implementing this pattern, lot of routine boiler plate code can be removed and code can be made more consistent.
I might make another blog post in the future on the usage of Func, Action and how Polly type of code can be written, and how some routine boiler plate code can be avoided.
For example, look at the following code:
try
{
DoSomething();
DoSomethingElse();
}
catch(Exception e)
{
logger.Error(message, e);
}
Instead we can implement something like this:
public static void Invoke(Action action, bool reThrow = false)
{
try {
action();
}
catch (Exception e) {
logger.LogError(e);
if (reThrow) throw;
}
}
Now the code can be something like:
Invoke(() => {
DoSomething();
DoSomethingElse();
});
For 2 lines of code, we wrote some boiler code and ended up with 9 lines of code i.e 7 lines of overhead code.
By using a Invoke() helper method, the overhead has been significantly reduced.
This pattern can be used in several situations.
–
Mr. Kanti Kalyan Arumilli
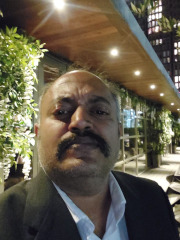
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
+44-33-3303-1284 (Preferred number if calling from U.K, No WhatsApp)
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.