In the past, I have written about a little known utility for collecting metrics known as CollectD. CollectD samples various metrics at configured interval and outputs to various destinations. This particular blog post is about having CollectD send the metrics to a GRPC endpoint, the endpoint can decide how to further process the received data. In this blog post, I would be writing about C# GRPC server for receiving data, but in reality most programming languages that support GRPC can be used.
One more thing, having CollectD use GRPC is slightly complex, because several different libraries need to be installed. Here is a list for Ubuntu, this is not an exhaustive list, but the list of libraries that I had to install on Ubuntu to allow CollectD report metrics using GRPC – gcc, gpp, build-essential, protobuf-compiler-grpc, libprotobuf-dev, protobuf-compiler, libgrpc++-dev. The best way to find any missing libraries is to compile CollectD from source as mentioned in https://collectd.org/download.shtml, and after ./configure look for missing libraries beside grpc until the output shows grpc – yes.
Now for the C# server, here is the .proto file, I have used:
syntax = "proto3";
package collectd;
import "google/protobuf/duration.proto";
import "google/protobuf/timestamp.proto";
service Collectd {
rpc PutValues(stream PutValuesRequest) returns(PutValuesResponse);
}
message PutValuesRequest {
ValueList value_list = 1;
}
message PutValuesResponse {}
message Identifier {
string host = 1;
string plugin = 2;
string plugin_instance = 3;
string type = 4;
string type_instance = 5;
}
message Value {
oneof value {
uint64 counter = 1;
double gauge = 2;
int64 derive = 3;
uint64 absolute = 4;
};
}
message ValueList {
repeated Value values = 1;
google.protobuf.Timestamp time = 2;
google.protobuf.Duration interval = 3;
Identifier identifier = 4;
repeated string ds_names = 5;
}
The .proto file is largely based on the proto files available at https://github.com/sandtable/collectd-grpc.
The implementation for the C# server is very simple. I have set the protobuf compiler to only generate the server side code. Create class that inherits from CollectdBase. Override the method PutValues. Remember the request is a stream.
public override async Task<PutValuesResponse> PutValues(IAsyncStreamReader<PutValuesRequest> requestStream, ServerCallContext context)
{
while (await requestStream.MoveNext())
{
var currentItem = requestStream.Current;
//Do something with currentItem
}
return new PutValuesResponse();
}
–
Mr. Kanti Kalyan Arumilli
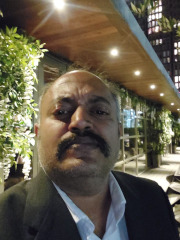
B.Tech, M.B.A
Founder & CEO, Lead Full-Stack .Net developer
ALight Technology And Services Limited
Phone / SMS / WhatsApp on the following 3 numbers:
+91-789-362-6688, +1-480-347-6849, +44-07718-273-964
kantikalyan@gmail.com, kantikalyan@outlook.com, admin@alightservices.com, kantikalyan.arumilli@alightservices.com, KArumilli2020@student.hult.edu, KantiKArumilli@outlook.com and 3 more rarely used email addresses – hardly once or twice a year.